本章需要掌握:
- 1、定义、初始化结构体、初始化结构体数组
- 2、什么是结构体对齐?结构体指针怎么用?指向结构体数组?指针偏移?
- 3、typedef重命名结构体
- 4、C++引用,再接往函数传入变量,函数拿到&变量,可以改变其值
1、结构体定义、初始化、结构体数组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| #include <stdio.h>
struct student{ int num; char name[20]; char sex; int age; float score; char addr[30]; };
int main() { struct student s={1001,"lele",'M',20,85.4,"Shenzhen"}; struct student sarr[3]; printf("%d %s %c %d %f %s\n",s.num,s.name,s.sex,s.age,s.score,s.addr); for(int i=0;i<3;i++) { scanf("%d%s %c%d%f%s",&sarr[i].num,sarr[i].name,&sarr[i].sex,&sarr[i].age, &sarr[i].score,sarr[i].addr); } for(int i=0;i<3;i++) { printf("%d %s %c %d %f %s\n",sarr[i].num,sarr[i].name,sarr[i].sex, sarr[i].age,sarr[i].score,sarr[i].addr); } return 0; }
|
2、结构体对齐
对齐是为了CPU高效的去取内存上的数据
结构体的大小必须是最大成员的整数倍,若两个相邻成员字节数只和小于最大成员则两个成员相当于一个最大成员的字节数。详细看下面注释。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include <stdio.h>
struct student_type1{ double score; short age; };
struct student_type2{ double score; int height; short age; };
struct student_type3{ int height; char sex; short age; };
|
3、结构体指针
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| #include <stdio.h>
struct student{ int num; char name[20]; char sex; };
int main() { struct student s={1001,"wangle",'M'}; struct student sarr[3]={1001,"lilei",'M',1005,"zhangsan",'M',1007,"lili",'F'}; struct student *p; p=&s; printf("%d %s %c\n",p->num,p->name,p->sex); p=sarr; printf("%d %s %c\n",(*p).num,(*p).name,(*p).sex); printf("%d %s %c\n",p->num,p->name,p->sex); p=p+1; printf("%d %s %c\n",p->num,p->name,p->sex); return 0; }
|
4、typedef 的使用
使用 typedef 声明新的类型名来代替已有的类型名
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| #include <stdio.h>
typedef struct student{ int num; char name[20]; char sex; }stu,*pstu;
typedef int INTEGER;
int main() { stu s={1001,"wangle",'M'}; pstu p; INTEGER i=10; p=&s; printf("i=%d,p->num=%d\n",i,p->num); return 0; }
|
5、C++引用讲解
如果想在子函数里面改变主函数的值,就加引用,不然就不加引用
注:这里是C++代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include <stdio.h>
void modify_num(int *b) { *b=*b+1; }
int main() { int a=10; modify_num(&a); printf("after modify_num a=%d\n",a);
int *p = &a; modify_num(p); printf("after modify_num a=%d\n",*p); return 0; }
|
二级指针(可以不懂)
先明确一点,*p指向NULL,不可访问(空指针不指向有效的内存区域);p指针指向[NULL的地址0] 。
* &p 是一个二级指针,&p拿到[p指针的地址],* &p拿到[p指向区域的地址值,即NULL的地址0] 。
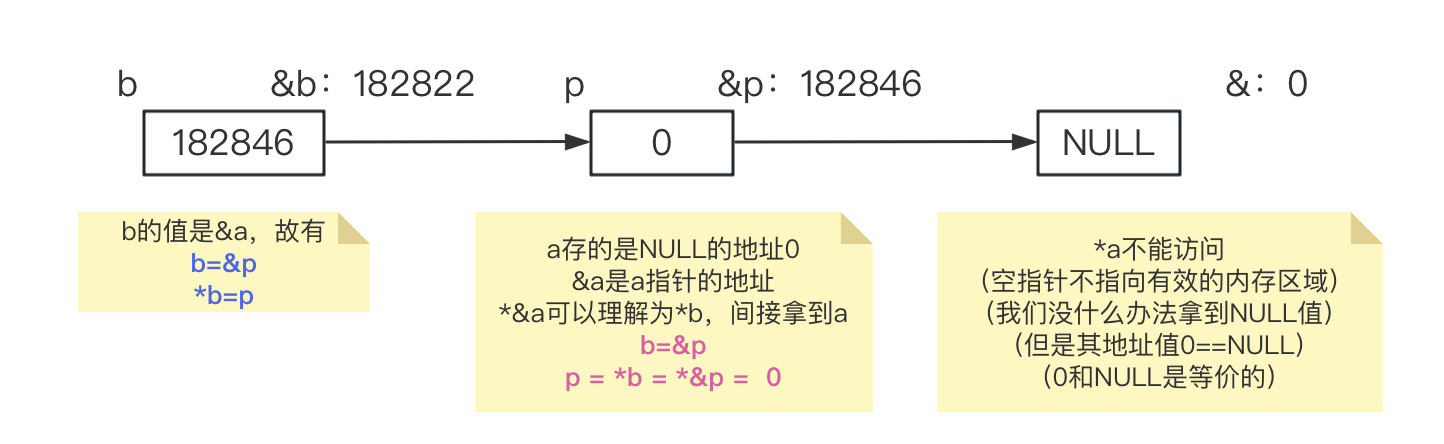
(在C\C++中,NULL等价于0,不用想这么多,测试可以换个数据测试)
(以数值为例,比如int i = 10;printf(“%d %d %d”,i,&i,*&i);,不难观察到i==*&i)
(以指针为例,比如int *p = &i;printf(“%d %d %d”,p,&p,*&p);,不难观察到p==*&p)
此处p不是值而是指针,p是NULL的地址0,*&p是指向&p地址的值NULL,NULL==0故二者等价)
这么说可能不直观,简单来说就是在下代码函数内修改p=q,即修改p指针内容0为q指针内容&i,借此p==q==&i,*p==*q==i。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| #include<iostream> using namespace std;
void modify_pointer(int* &p, int* q) { p = q; }
int main() { int *p = NULL; int i = 10; int* q = &i; modify_pointer(p, q); if (p != NULL) { printf("after modify_pointer *p=%d\n", *p); } return 0; }
|
🌺6、OJ作业
网站:http://oj.lgwenda.com/
1、结构体(struct)
描述:输入一个学生的学号,姓名,性别,用结构体存储,通过scanf读取后,然后再通过printf打印输出
输入:学号,姓名,性别,例如输入 101 xiongda m
输出:输出和输入的内容一致,如果输入的是101 xiongda m,那么输出也是101 xiongda m
🌺🌺🌺题解ヾ(^▽^)ゞ🌼🌼🌼
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| #include "stdio.h"
struct student{ int num; char name[20]; char sex; };
int main(){ struct student s; scanf("%d %s %c",&s.num,&s.name,&s.sex); printf("%d %s %c",s.num,s.name,s.sex); return 0; }
|
2、C++引用(quote)
描述:使用C++的引用,注意提交时把代码选为C++;在主函数定义字符指针 char *p,然后在子函数内malloc申请空间(大小为100个字节),通过fgets读取字符串,然后在主函数中进行输出;要求子函数使用C++的引用,注意在C++中从标准输入读取字符串,需要使用fgets(p,100,stdin)
输入:输入一个字符串,例如 I love C language
输出:如果输入的是I love C language,那么输出也是I love C language
🌺🌺🌺题解ヾ(^▽^)ゞ🌼🌼🌼
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| #include <stdio.h> #include <stdlib.h>
void modify_pointer(char *&p) { p=(char*)malloc(100); fgets(p,100,stdin); }
int main() { char *p=NULL; modify_pointer(p); puts(p); free(p); return 0; }
|